
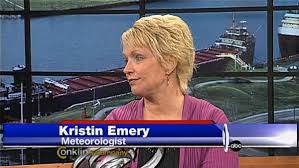
In main, once all the customer threads have been joined, it sets finished to true, and wakes up the (possibly) sleeping Barber to finish the program.īy this method, both the Barber and the Customer read chairs first followed by the status, and release it in the opposite order (preventing any deadlocks from circular waiting).Classic IPC problem using threads in C. Print("Customer is finished getting a haircut") Print("Barber Shop is busy, will return later") Helped = true // This customer is being helped Wait(waiting) # Be the next customer to get helped (happens immediately) Print("Customer arrived at the Barber Shop!") Print("Barber finished work for the day") Signal(cutting) # Release the customer so they can leave Print("Barber finished cutting the customer's hair") Print("Barber is cutting a customer's hair") # Once it gets here, the barber is awake and must help someone Signal(waiting) # Let the next customer in # If there is nobody waiting and the barber isn't asleep Int status = AWAKE # Barber Status (could also be a boolean for sleeping)īool finished = false # Is the program finished Int chairs = n # Available chairs to sit in Semaphore sleeping = 0 # Semaphore for barber to sleep Semaphore cutting = 0 # Semaphore to hold customer while getting hair cut Semaphore waiting = 0 # Line for waiting customers Semaphore rw_status = 1 # Access to barber status Semaphore rw_chairs = 1 # Access to chairs The pseudocode is the following: # Constants Instead of using 6 semaphores, I only used 5: 2 that control read/write access, and 3 that control queueing/sleeping. My original method wasn't entirely off, however there were some minor tweaks that were needed to get it to work properly. Seeing as I haven't seen this asked anywhere on here before, I will answer my own question in case anyone else comes across this looking for help like I did. Or if I'm on the right track, possibly a push in the right direction.
#SLEEPING BARBER PROGRAM IN C HOW TO#
If anyone here has any suggestions for how to build a working solution, or suggestions on restructuring my current one, that'd be greatly appreciated. I think I'm approaching the problem wrong, and likely overcomplicating it. Its clear to me that the barber isn't properly letting a customer in - but even in that I feel like my structure is all wrong.

When this doesn't get a deadlock, using 3 chairs I get the following output: Barber has fallen asleep Print("Customer is leaving the barbershop") Print("All chairs taken, will return later") Wait(cutting) # Wait for him to finish cutting hair Wait(barber_ready) # Wait for the barber to be ready Print("Customer has woken the barber up")
#SLEEPING BARBER PROGRAM IN C FREE#
If chairs = N and not is_cutting: # If the barber is free Signal(cutting) # Let the customer leave after the hair cut # If the barber isn't done for the day, help the customer

Signal(barber_ready) # Allow customers into the chair Wait(sleeping) # Wait for customer to wake him up Signal(rw_chairs) # Allow others to read/write Semaphore cutting = 0 # Barber cutting a customer's hair Semaphore sleeping = 0 # Barber is sleeping Semaphore barber_ready = 0 # Barber ready to cut hair My current solution, in pseudocode, is something like this: int chairs = N # available chairsīool finished = false # All customers taken care of (Changed in main) "Barber has fallen asleep" followed by "customer has woken the barber up") or it would print statements out of order as a result of a context switch (which may or may not be a real issue). The classical solution for the problem wouldn't fully apply here because it would result in statements either being printed every time (e.g. I've been working at this problem for a little bit, only to get various deadlocks or having statements printed out-of-order. Customer left because no seats were available (only to return a random period of time later).I'm trying to implement a solution to the sleeping barber problem in C using pthreads and semaphores, only part of the requirement is that each action has to be printed out when it happens, such as:
